原生组件
如果你想构建一个 新架构 的 React Native 组件,该组件可以包装一个 Host Component,例如 Android 上的 CheckBox,或者 iOS 上的 UIButton,你应该使用 Fabric 原生组件。
本指南将以实现一个 web 视图组件为例,展示如何构建 Fabric 原生组件。步骤如下:
- 使用 Flow 或 TypeScript 定义一个 JavaScript 规范。
- 配置依赖管理系统的代码生成功能,并自动链接。
- 实现原生代码。
- 在应用中使用该组件。
你需要一个普通的模板生成应用来使用该组件:
npx @react-native-community/cli@latest init Demo --install-pods false
创建一个 WebView 组件
本指南将展示如何创建一个 Web View 组件。我们将使用 Android 的 WebView
组件和 iOS 的 WKWebView
组件来创建该组件。
首先,创建一个文件夹结构来存放组件的代码:
mkdir -p Demo/{specs,android/app/src/main/java/com/webview}
这将创建以下布局,你将在其中工作:
Demo
├── android/app/src/main/java/com/webview
└── ios
└── spec
android/app/src/main/java/com/webview
文件夹是存放 Android 代码的文件夹。ios
文件夹是存放 iOS 代码的文件夹。spec
文件夹是存放 Codegen 规范文件的文件夹。
1. 定义 Codegen 规范
你的规范必须使用 TypeScript 或 Flow 定义(更多详情请参阅 Codegen 文档)。这是由 Codegen 生成 C++、Objective-C++ 和 Java 代码,以连接你的平台代码到 React 运行的 JavaScript 运行时。
规范文件必须命名为 <MODULE_NAME>NativeComponent.{ts|js}
才能被 Codegen 识别。NativeComponent
后缀不仅是一个约定,实际上是由 Codegen 用于检测规范文件。
使用以下规范文件来创建 WebView 组件:
- TypeScript
- Flow
import type {HostComponent, ViewProps} from 'react-native';
import type {BubblingEventHandler} from 'react-native/Libraries/Types/CodegenTypes';
import codegenNativeComponent from 'react-native/Libraries/Utilities/codegenNativeComponent';
type WebViewScriptLoadedEvent = {
result: 'success' | 'error';
};
export interface NativeProps extends ViewProps {
sourceURL?: string;
onScriptLoaded?: BubblingEventHandler<WebViewScriptLoadedEvent> | null;
}
export default codegenNativeComponent<NativeProps>(
'CustomWebView',
) as HostComponent<NativeProps>;
// @flow strict-local
import type {HostComponent, ViewProps} from 'react-native';
import type {BubblingEventHandler} from 'react-native/Libraries/Types/CodegenTypes';
import codegenNativeComponent from 'react-native/Libraries/Utilities/codegenNativeComponent';
type WebViewScriptLoadedEvent = $ReadOnly<{|
result: "success" | "error",
|}>;
type NativeProps = $ReadOnly<{|
...ViewProps,
sourceURL?: string;
onScriptLoaded?: BubblingEventHandler<WebViewScriptLoadedEvent>?;
|}>;
export default (codegenNativeComponent<NativeProps>(
'CustomWebView',
): HostComponent<NativeProps>);
该规范文件由三部分组成,不包括导入:
WebViewScriptLoadedEvent
是一个支持的数据类型,用于将数据从原生代码传递到 JavaScript。NativeProps
是定义可以在组件上设置的属性。codegenNativeComponent
语句允许我们为自定义组件生成代码,并定义用于匹配原生实现的名称。
与原生模块一样,你可以在 specs/
目录中拥有多个规范文件。更多信息请参阅 附录。
2. 配置 Codegen 运行
该规范文件用于 React Native 的 Codegen 工具生成平台特定的接口和样板代码。为此,Codegen 需要知道在哪里找到我们的规范文件以及如何处理它。更新你的 package.json
文件:
"start": "react-native start",
"test": "jest"
},
"codegenConfig": {
"name": "AppSpec",
"type": "components",
"jsSrcsDir": "specs",
"android": {
"javaPackageName": "com.webview"
}
},
"dependencies": {
配置好 Codegen 后,我们需要准备原生代码以连接到生成的代码。
2. 构建原生代码
现在,是时候编写原生平台代码,以便当 React 需要渲染视图时,平台可以创建正确的原生视图并在屏幕上渲染它。
你应该分别在 Android 和 iOS 平台上工作。
本指南展示了如何创建一个仅适用于新架构的原生组件。如果你需要同时支持新架构和旧架构,请参阅我们的向后兼容指南。
- Android
- iOS
现在,是时候编写一些 Android 平台代码,以便能够渲染 web 视图。以下是需要的步骤:
- 运行 Codegen
- 编写
ReactWebView
的代码 - 编写
ReactWebViewManager
的代码 - 编写
ReactWebViewPackage
的代码 - 在应用中注册
ReactWebViewPackage
1. 使用 Gradle 运行 Codegen
运行一次以生成你的 IDE 可以使用的样板代码。
cd android
./gradlew generateCodegenArtifactsFromSchema
Codegen 将生成你需要实现 ViewManager
接口和 ViewManager
委托的 web 视图。
2. 编写 ReactWebView
ReactWebView
是包装 Android 原生视图的组件,React Native 将在使用自定义组件时渲染它。
在 android/src/main/java/com/webview
文件夹中创建一个 ReactWebView.java
或 ReactWebView.kt
文件,并使用以下代码:
- Java
- Kotlin
package com.webview;
import android.content.Context;
import android.util.AttributeSet;
import android.webkit.WebView;
import android.webkit.WebViewClient;
import com.facebook.react.bridge.Arguments;
import com.facebook.react.bridge.WritableMap;
import com.facebook.react.bridge.ReactContext;
import com.facebook.react.uimanager.UIManagerHelper;
import com.facebook.react.uimanager.events.Event;
public class ReactWebView extends WebView {
public ReactWebView(Context context) {
super(context);
configureComponent();
}
public ReactWebView(Context context, AttributeSet attrs) {
super(context, attrs);
configureComponent();
}
public ReactWebView(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
configureComponent();
}
private void configureComponent() {
this.setLayoutParams(new LayoutParams(LayoutParams.MATCH_PARENT, LayoutParams.MATCH_PARENT));
this.setWebViewClient(new WebViewClient() {
@Override
public void onPageFinished(WebView view, String url) {
emitOnScriptLoaded(OnScriptLoadedEventResult.success);
}
});
}
public void emitOnScriptLoaded(OnScriptLoadedEventResult result) {
ReactContext reactContext = (ReactContext) context;
int surfaceId = UIManagerHelper.getSurfaceId(reactContext);
EventDispatcher eventDispatcher = UIManagerHelper.getEventDispatcherForReactTag(reactContext, getId());
WritableMap payload = Arguments.createMap();
payload.putString("result", result.name());
OnScriptLoadedEvent event = new OnScriptLoadedEvent(surfaceId, getId(), payload);
if (eventDispatcher != null) {
eventDispatcher.dispatchEvent(event);
}
}
public enum OnScriptLoadedEventResult {
success,
error
}
private class OnScriptLoadedEvent extends Event<OnScriptLoadedEvent> {
private final WritableMap payload;
OnScriptLoadedEvent(int surfaceId, int viewId, WritableMap payload) {
super(surfaceId, viewId);
this.payload = payload;
}
@Override
public String getEventName() {
return "onScriptLoaded";
}
@Override
public WritableMap getEventData() {
return payload;
}
}
}
package com.webview
import android.content.Context
import android.util.AttributeSet
import android.webkit.WebView
import android.webkit.WebViewClient
import com.facebook.react.bridge.Arguments
import com.facebook.react.bridge.WritableMap
import com.facebook.react.bridge.ReactContext
import com.facebook.react.uimanager.UIManagerHelper
import com.facebook.react.uimanager.events.Event
class ReactWebView: WebView {
constructor(context: Context) : super(context) {
configureComponent()
}
constructor(context: Context, attrs: AttributeSet?) : super(context, attrs) {
configureComponent()
}
constructor(context: Context, attrs: AttributeSet?, defStyleAttr: Int) : super(context, attrs, defStyleAttr) {
configureComponent()
}
private fun configureComponent() {
this.layoutParams = LayoutParams(LayoutParams.MATCH_PARENT, LayoutParams.MATCH_PARENT)
this.webViewClient = object : WebViewClient() {
override fun onPageFinished(view: WebView, url: String) {
emitOnScriptLoaded(OnScriptLoadedEventResult.success)
}
}
}
fun emitOnScriptLoaded(result: OnScriptLoadedEventResult) {
val reactContext = context as ReactContext
val surfaceId = UIManagerHelper.getSurfaceId(reactContext)
val eventDispatcher = UIManagerHelper.getEventDispatcherForReactTag(reactContext, id)
val payload =
Arguments.createMap().apply {
putString("result", result.name)
}
val event = OnScriptLoadedEvent(surfaceId, id, payload)
eventDispatcher?.dispatchEvent(event)
}
enum class OnScriptLoadedEventResult() {
success(),
error()
}
inner class OnScriptLoadedEvent(
surfaceId: Int,
viewId: Int,
private val payload: WritableMap
) : Event<OnScriptLoadedEvent>(surfaceId, viewId) {
override fun getEventName() = "onScriptLoaded"
override fun getEventData() = payload
}
}
ReactWebView
扩展了 Android WebView
,因此你可以轻松地重用平台已经定义的所有属性。
该类定义了三个 Android 构造函数,但将它们的实际实现推迟到私有 configureComponent
函数。此函数负责初始化所有组件的特定属性:在这种情况下,你正在设置 WebView
的布局,并定义你用于自定义 WebView
行为的 WebClient
。在此代码中,ReactWebView
在页面加载完成后通过实现 WebClient
的 onPageFinished
方法来发出事件。
然后,代码定义了一个实际发出事件的帮助函数。要发出事件,你必须:
- 获取
ReactContext
的引用; - retrieve the
surfaceId
of the view that you are presenting; - grab a reference to the
eventDispatcher
associated with the view; - build the payload for the event using a
WritableMap
object; - create the event object that you need to send to JavaScript;
- call the
eventDispatcher.dispatchEvent
to send the event.
The last part of the file contains the definition of the data types you need to send the event:
- The
OnScriptLoadedEventResult
, with the possible outcomes of theOnScriptLoaded
event. - The actual ``OnScriptLoadedEvent
that needs to extend the React Native's
Event` class.
3. Write the WebViewManager
The WebViewManager
is the class that connects the React Native runtime with the native view.
When React receives the instruction from the app to render a specific component, React uses the registered view manager to create the view and to pass all the required properties.
This is the code of the ReactWebViewManager
.
- Java
- Kotlin
package com.webview;
import com.facebook.react.bridge.ReactApplicationContext;
import com.facebook.react.module.annotations.ReactModule;
import com.facebook.react.uimanager.SimpleViewManager;
import com.facebook.react.uimanager.ThemedReactContext;
import com.facebook.react.uimanager.ViewManagerDelegate;
import com.facebook.react.uimanager.annotations.ReactProp;
import com.facebook.react.viewmanagers.CustomWebViewManagerInterface;
import com.facebook.react.viewmanagers.CustomWebViewManagerDelegate;
import java.util.HashMap;
import java.util.Map;
@ReactModule(name = ReactWebViewManager.REACT_CLASS)
class ReactWebViewManager extends SimpleViewManager<ReactWebView> implements CustomWebViewManagerInterface<ReactWebView> {
private final CustomWebViewManagerDelegate<ReactWebView, ReactWebViewManager> delegate =
new CustomWebViewManagerDelegate<>(this);
@Override
public ViewManagerDelegate<ReactWebView> getDelegate() {
return delegate;
}
@Override
public String getName() {
return REACT_CLASS;
}
@Override
public ReactWebView createViewInstance(ThemedReactContext context) {
return new ReactWebView(context);
}
@ReactProp(name = "sourceUrl")
@Override
public void setSourceURL(ReactWebView view, String sourceURL) {
if (sourceURL == null) {
view.emitOnScriptLoaded(ReactWebView.OnScriptLoadedEventResult.error);
return;
}
view.loadUrl(sourceURL, new HashMap<>());
}
public static final String REACT_CLASS = "CustomWebView";
@Override
public Map<String, Object> getExportedCustomBubblingEventTypeConstants() {
Map<String, Object> map = new HashMap<>();
Map<String, Object> bubblingMap = new HashMap<>();
bubblingMap.put("phasedRegistrationNames", new HashMap<String, String>() {{
put("bubbled", "onScriptLoaded");
put("captured", "onScriptLoadedCapture");
}});
map.put("onScriptLoaded", bubblingMap);
return map;
}
}
package com.webview
import com.facebook.react.bridge.ReactApplicationContext;
import com.facebook.react.module.annotations.ReactModule;
import com.facebook.react.uimanager.SimpleViewManager;
import com.facebook.react.uimanager.ThemedReactContext;
import com.facebook.react.uimanager.ViewManagerDelegate;
import com.facebook.react.uimanager.annotations.ReactProp;
import com.facebook.react.viewmanagers.CustomWebViewManagerInterface;
import com.facebook.react.viewmanagers.CustomWebViewManagerDelegate;
@ReactModule(name = ReactWebViewManager.REACT_CLASS)
class ReactWebViewManager(context: ReactApplicationContext) : SimpleViewManager<ReactWebView>(), CustomWebViewManagerInterface<ReactWebView> {
private val delegate: CustomWebViewManagerDelegate<ReactWebView, ReactWebViewManager> =
CustomWebViewManagerDelegate(this)
override fun getDelegate(): ViewManagerDelegate<ReactWebView> = delegate
override fun getName(): String = REACT_CLASS
override fun createViewInstance(context: ThemedReactContext): ReactWebView = ReactWebView(context)
@ReactProp(name = "sourceUrl")
override fun setSourceURL(view: ReactWebView, sourceURL: String?) {
if (sourceURL == null) {
view.emitOnScriptLoaded(ReactWebView.OnScriptLoadedEventResult.error)
return;
}
view.loadUrl(sourceURL, emptyMap())
}
companion object {
const val REACT_CLASS = "CustomWebView"
}
override fun getExportedCustomBubblingEventTypeConstants(): Map<String, Any> =
mapOf(
"onScriptLoaded" to
mapOf(
"phasedRegistrationNames" to
mapOf(
"bubbled" to "onScriptLoaded",
"captured" to "onScriptLoadedCapture"
)))
}
The ReactWebViewManager
extends the SimpleViewManager
class from React and implements the CustomWebViewManagerInterface
, generated by Codegen.
It holds a reference of the CustomWebViewManagerDelegate
, another element generated by Codegen.
It then overrides the getName
function, which must return the same name used in the spec's codegenNativeComponent
function call.
The createViewInstance
function is responsible to instantiate a new ReactWebView
.
Then, the ViewManager needs to define how all the React's compnoents props will update the native view. In the example, you need to decide how to handle the sourceURL
property that React will set on the WebView
.
Finally, if the component can emit an event, you need to map the event name by overriding the getExportedCustomBubblingEventTypeConstants
for bubbling events, or the getExportedCustomDirectEventTypeConstants
for direct events.
4. Write the ReactWebViewPackage
As you do with Native Modules, Native Components also need to implement the ReactPackage
class. This is an object that you can use to register the component in the React Native runtime.
This is the code for the ReactWebViewPackage
:
- Java
- Kotlin
package com.webview;
import com.facebook.react.TurboReactPackage;
import com.facebook.react.bridge.NativeModule;
import com.facebook.react.bridge.ReactApplicationContext;
import com.facebook.react.module.model.ReactModuleInfo;
import com.facebook.react.module.model.ReactModuleInfoProvider;
import com.facebook.react.uimanager.ViewManager;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class ReactWebViewPackage extends TurboReactPackage {
@Override
public List<ViewManager<?, ?>> createViewManagers(ReactApplicationContext reactContext) {
return Collections.singletonList(new ReactWebViewManager(reactContext));
}
@Override
public NativeModule getModule(String s, ReactApplicationContext reactApplicationContext) {
if (ReactWebViewManager.REACT_CLASS.equals(s)) {
return new ReactWebViewManager(reactApplicationContext);
}
return null;
}
@Override
public ReactModuleInfoProvider getReactModuleInfoProvider() {
return new ReactModuleInfoProvider() {
@Override
public Map<String, ReactModuleInfo> get() {
Map<String, ReactModuleInfo> map = new HashMap<>();
map.put(ReactWebViewManager.REACT_CLASS, new ReactModuleInfo(
ReactWebViewManager.REACT_CLASS, // name
ReactWebViewManager.REACT_CLASS, // className
false, // canOverrideExistingModule
false, // needsEagerInit
false, // isCxxModule
true // isTurboModule
));
return map;
}
};
}
}
package com.webview
import com.facebook.react.TurboReactPackage
import com.facebook.react.bridge.NativeModule
import com.facebook.react.bridge.ReactApplicationContext
import com.facebook.react.module.model.ReactModuleInfo
import com.facebook.react.module.model.ReactModuleInfoProvider
import com.facebook.react.uimanager.ViewManager
class ReactWebViewPackage : TurboReactPackage() {
override fun createViewManagers(reactContext: ReactApplicationContext): List<ViewManager<*, *>> {
return listOf(ReactWebViewManager(reactContext))
}
override fun getModule(s: String, reactApplicationContext: ReactApplicationContext): NativeModule? {
when (s) {
ReactWebViewManager.REACT_CLASS -> ReactWebViewManager(reactApplicationContext)
}
return null
}
override fun getReactModuleInfoProvider(): ReactModuleInfoProvider = ReactModuleInfoProvider {
mapOf(ReactWebViewManager.REACT_CLASS to ReactModuleInfo(
_name = ReactWebViewManager.REACT_CLASS,
_className = ReactWebViewManager.REACT_CLASS,
_canOverrideExistingModule = false,
_needsEagerInit = false,
isCxxModule = false,
isTurboModule = true,
)
)
}
}
The ReactWebViewPackage
extends the TurboReactPackage
and implements all the methods required to properly register our component.
- the
createViewManagers
method is the factory method that creates theViewManager
that manage the custom views. - the
getModule
method returns the proper ViewManager depending on the View that React Native needs to render. - the
getReactModuleInfoProvider
provides all the information required when registering the module in the runtime,
5. Register the ReactWebViewPackage
in the application
Finally, you need to register the ReactWebViewPackage
in the application. We do that by modifying the MainApplication
file by adding the ReactWebViewPackage
to the list of packages returned by the getPackages
function.
package com.demo
import android.app.Application
import com.facebook.react.PackageList
import com.facebook.react.ReactApplication
import com.facebook.react.ReactHost
import com.facebook.react.ReactNativeHost
import com.facebook.react.ReactPackage
import com.facebook.react.defaults.DefaultNewArchitectureEntryPoint.load
import com.facebook.react.defaults.DefaultReactHost.getDefaultReactHost
import com.facebook.react.defaults.DefaultReactNativeHost
import com.facebook.react.soloader.OpenSourceMergedSoMapping
import com.facebook.soloader.SoLoader
import com.webview.ReactWebViewPackage
class MainApplication : Application(), ReactApplication {
override val reactNativeHost: ReactNativeHost =
object : DefaultReactNativeHost(this) {
override fun getPackages(): List<ReactPackage> =
PackageList(this).packages.apply {
add(ReactWebViewPackage())
}
override fun getJSMainModuleName(): String = "index"
override fun getUseDeveloperSupport(): Boolean = BuildConfig.DEBUG
override val isNewArchEnabled: Boolean = BuildConfig.IS_NEW_ARCHITECTURE_ENABLED
override val isHermesEnabled: Boolean = BuildConfig.IS_HERMES_ENABLED
}
override val reactHost: ReactHost
get() = getDefaultReactHost(applicationContext, reactNativeHost)
override fun onCreate() {
super.onCreate()
SoLoader.init(this, OpenSourceMergedSoMapping)
if (BuildConfig.IS_NEW_ARCHITECTURE_ENABLED) {
load()
}
}
}
现在,是时候编写一些 iOS 平台代码,以便能够渲染 web 视图。以下是需要的步骤:
- 运行 Codegen。
- 编写
RCTWebView
的代码 - 在应用中注册
RCTWebView
1. 运行 Codegen
你可以手动运行 Codegen,当然直接使用你将要演示组件的应用来完成此操作会更简单。
cd ios
bundle install
bundle exec pod install
重要的是你会看到 Codegen 的日志输出,我们将在 Xcode 中使用它来构建 WebView 原生组件。
注意不要将生成的代码提交到你的仓库中。生成的代码与 React Native 的版本相关。使用 npm peerDependencies 来约束与 React Native 版本的兼容性。
3. 编写 RCTWebView
我们需要使用 Xcode 完成以下 5 步:
- 打开 CocoPods 生成的 Xcode workspace 文件:
cd ios
open Demo.xcworkspace
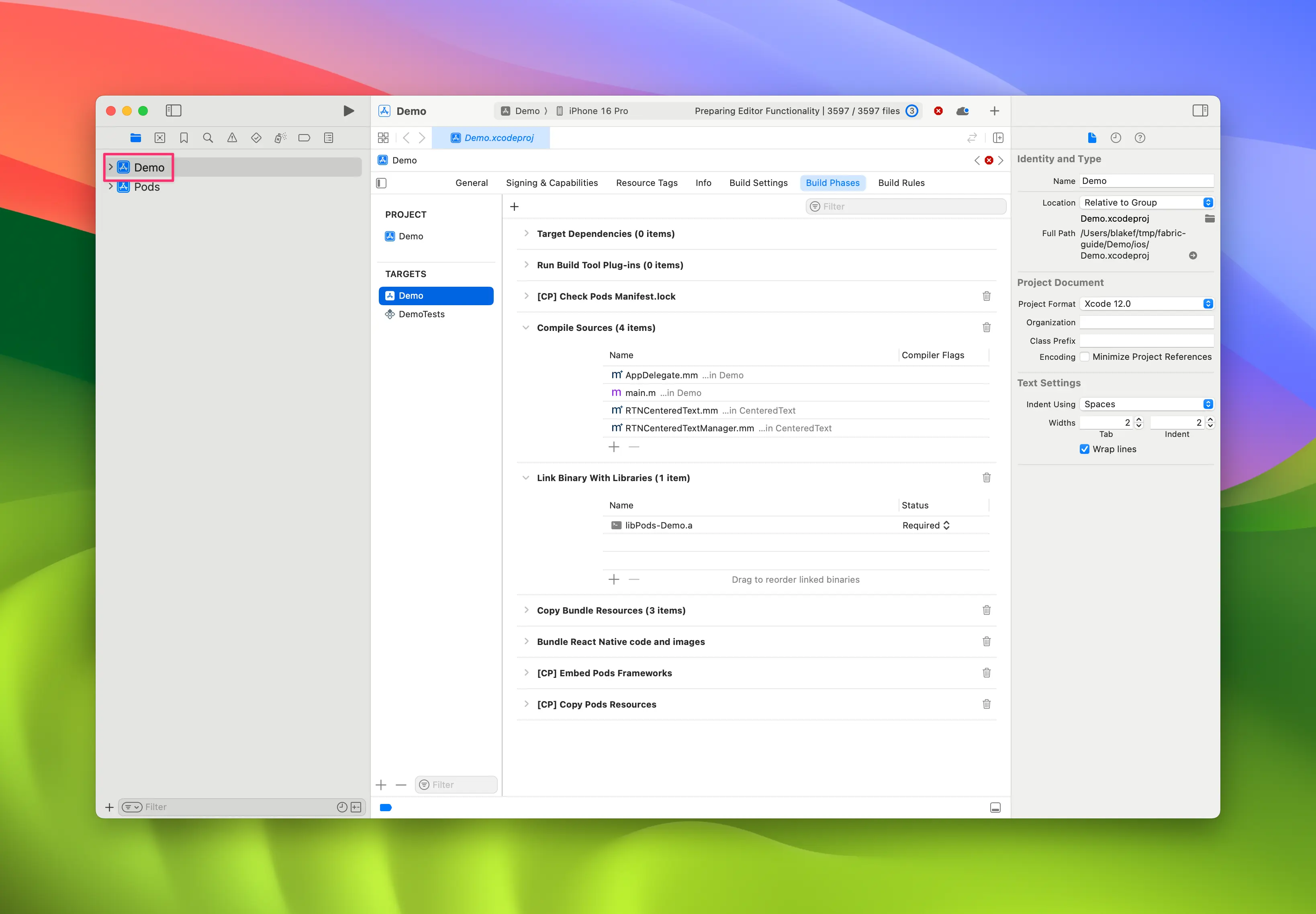
- 右键点击应用,选择
New Group
,将新组命名为WebView
。
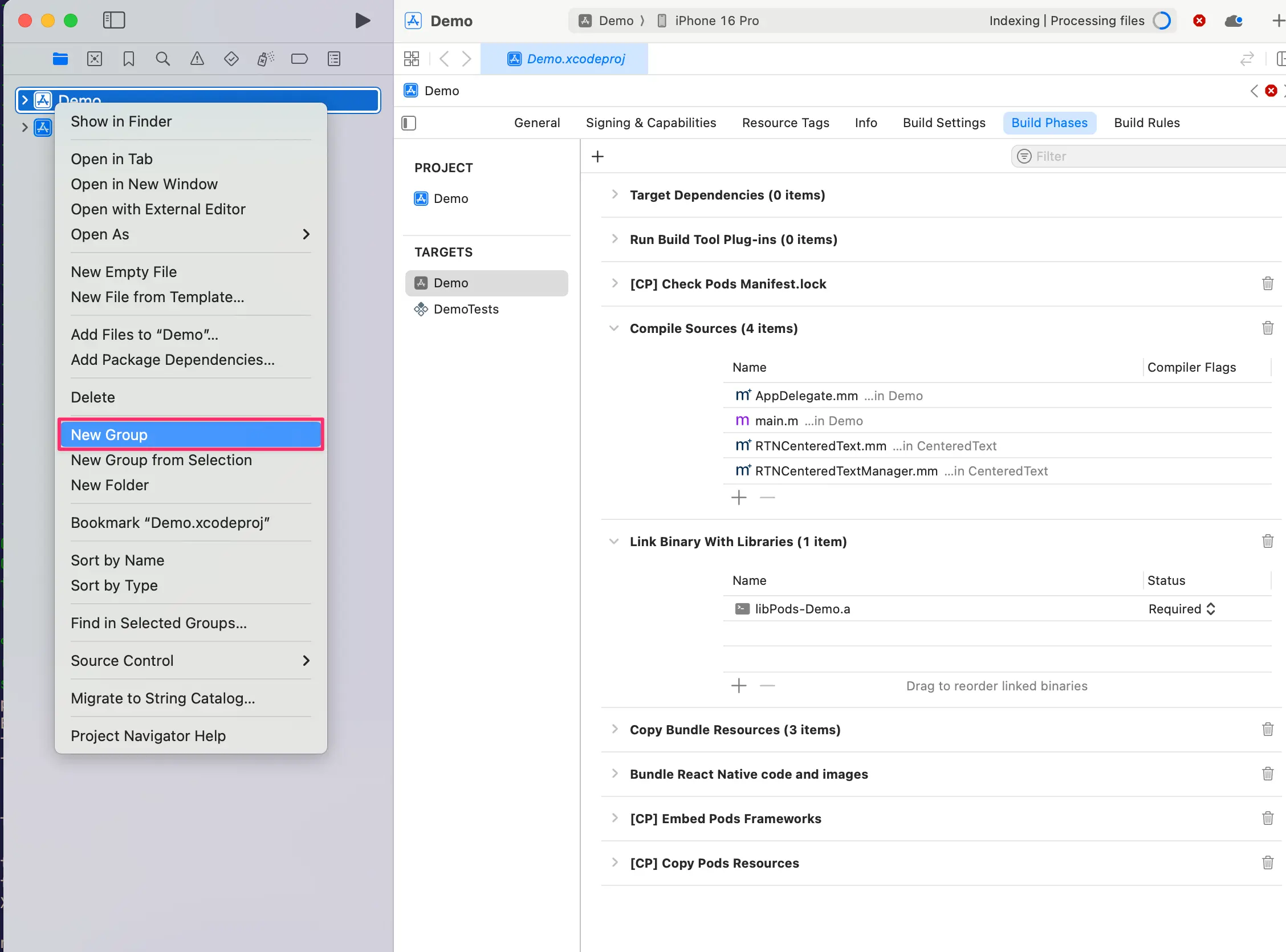
- 在
WebView
组中,创建New
→File from Template
。
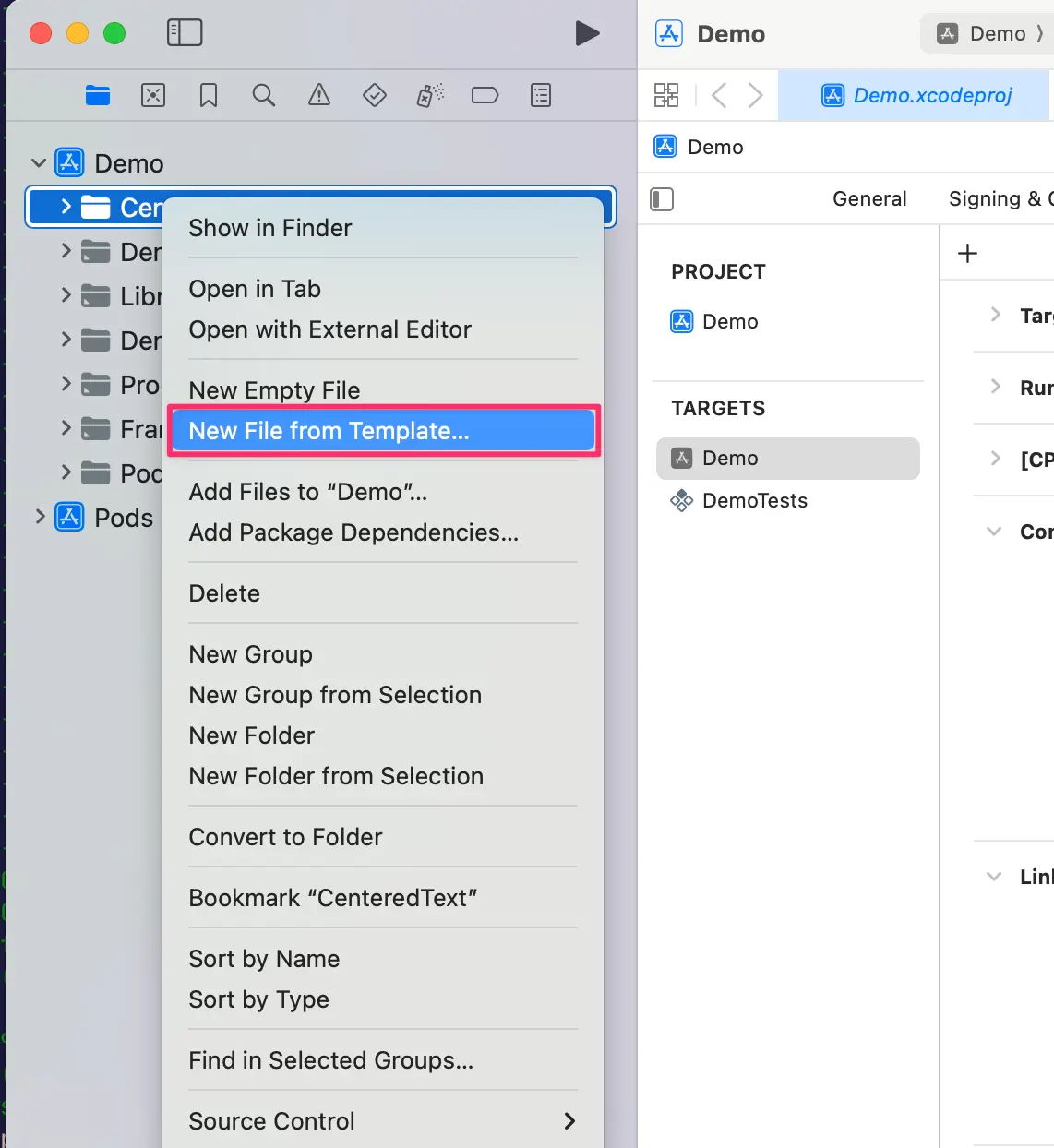
- 使用
Objective-C File
模板,并命名为RCTWebView
。
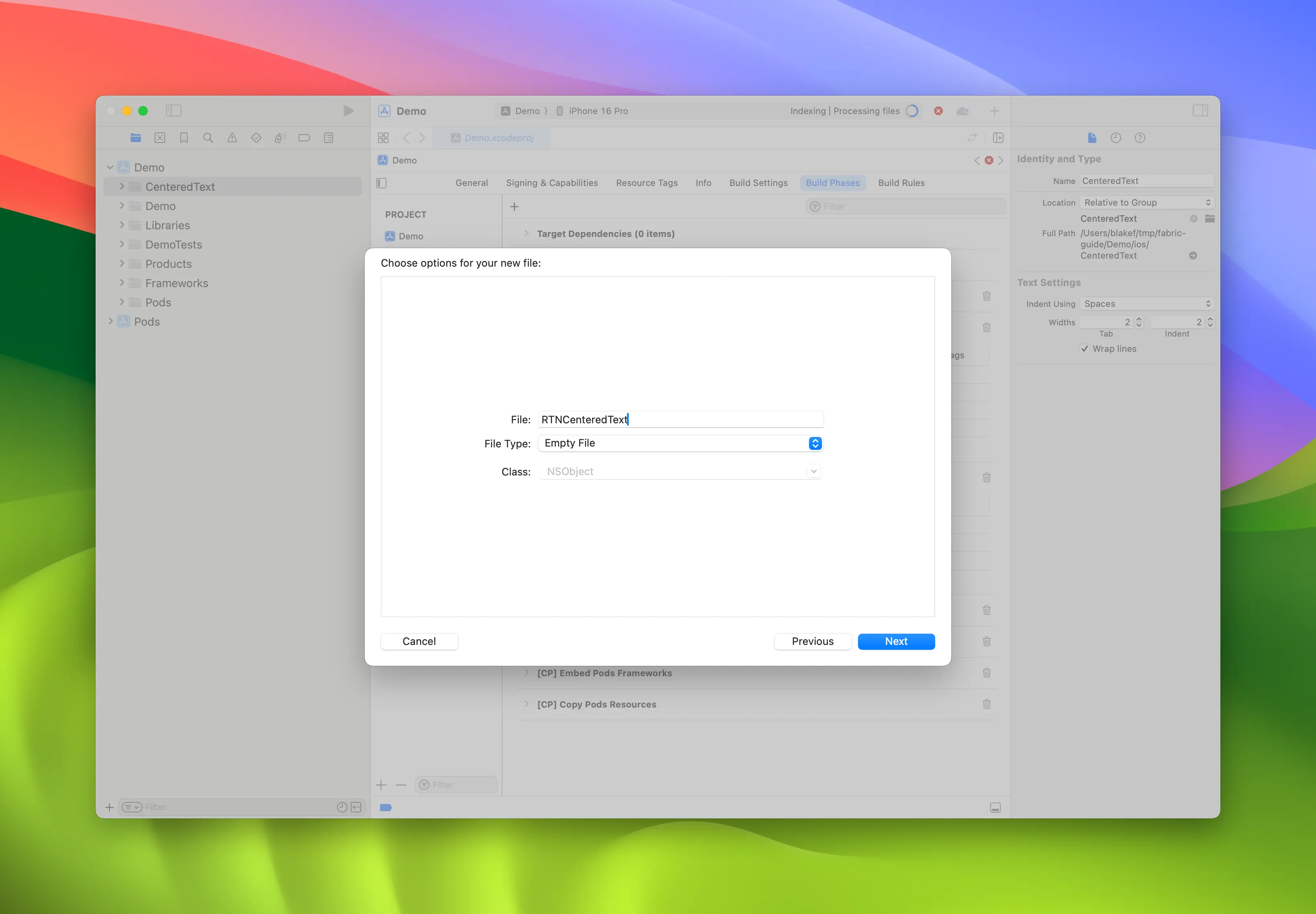
- 将
RCTWebView.m
重命名为RCTWebView.mm
,使其成为 Objective-C++ 文件。
Podfile
...
Demo
├── AppDelegate.h
├── AppDelegate.mm
...
├── RCTWebView.h
├── RCTWebView.mm
└── main.m
创建头文件和实现文件后,可以开始实现它们。
以下是 RCTWebView.h
文件的代码,声明了组件接口。
#import <React/RCTViewComponentView.h>
#import <UIKit/UIKit.h>
NS_ASSUME_NONNULL_BEGIN
@interface RCTWebView : RCTViewComponentView
// 你可以在视图中声明你想要访问的原生方法
@end
NS_ASSUME_NONNULL_END
这个类定义了一个 RCTWebView
,它扩展了 RCTViewComponentView
类。这是所有原生组件的基础类,由 React Native 提供。
以下是实现文件 (RCTWebView.mm
) 的代码:
#import "RCTWebView.h"
#import <react/renderer/components/AppSpecs/ComponentDescriptors.h>
#import <react/renderer/components/AppSpecs/EventEmitters.h>
#import <react/renderer/components/AppSpecs/Props.h>
#import <react/renderer/components/AppSpecs/RCTComponentViewHelpers.h>
#import <WebKit/WebKit.h>
using namespace facebook::react;
@interface RCTWebView () <RCTCustomWebViewViewProtocol, WKNavigationDelegate>
@end
@implementation RCTWebView {
NSURL * _sourceURL;
WKWebView * _webView;
}
-(instancetype)init
{
if(self = [super init]) {
_webView = [WKWebView new];
_webView.navigationDelegate = self;
[self addSubview:_webView];
}
return self;
}
- (void)updateProps:(Props::Shared const &)props oldProps:(Props::Shared const &)oldProps
{
const auto &oldViewProps = *std::static_pointer_cast<CustomWebViewProps const>(_props);
const auto &newViewProps = *std::static_pointer_cast<CustomWebViewProps const>(props);
// Handle your props here
if (oldViewProps.sourceURL != newViewProps.sourceURL) {
NSString *urlString = [NSString stringWithCString:newViewProps.sourceURL.c_str() encoding:NSUTF8StringEncoding];
_sourceURL = [NSURL URLWithString:urlString];
if ([self urlIsValid:newViewProps.sourceURL]) {
[_webView loadRequest:[NSURLRequest requestWithURL:_sourceURL]];
}
}
[super updateProps:props oldProps:oldProps];
}
-(void)layoutSubviews
{
[super layoutSubviews];
_webView.frame = self.bounds;
}
#pragma mark - WKNavigationDelegate
-(void)webView:(WKWebView *)webView didFinishNavigation:(WKNavigation *)navigation
{
CustomWebViewEventEmitter::OnScriptLoaded result = CustomWebViewEventEmitter::OnScriptLoaded{CustomWebViewEventEmitter::OnScriptLoadedResult::Success};
self.eventEmitter.onScriptLoaded(result);
}
- (BOOL)urlIsValid:(std::string)propString
{
if (propString.length() > 0 && !_sourceURL) {
CustomWebViewEventEmitter::OnScriptLoaded result = CustomWebViewEventEmitter::OnScriptLoaded{CustomWebViewEventEmitter::OnScriptLoadedResult::Error};
self.eventEmitter.onScriptLoaded(result);
return NO;
}
return YES;
}
// Event emitter convenience method
- (const CustomWebViewEventEmitter &)eventEmitter
{
return static_cast<const CustomWebViewEventEmitter &>(*_eventEmitter);
}
+ (ComponentDescriptorProvider)componentDescriptorProvider
{
return concreteComponentDescriptorProvider<CustomWebViewComponentDescriptor>();
}
Class<RCTComponentViewProtocol> WebViewCls(void)
{
return RCTWebView.class;
}
@end
这段代码是用 Objective-C++ 编写的,包含以下细节:
@interface
实现了两个协议:RCTCustomWebViewViewProtocol
, generated by Codegen;WKNavigationDelegate
, 由 WebKit 框架提供,用于处理 web 视图导航事件;
init
方法实例化WKWebView
,将其添加到子视图,并设置navigationDelegate
;updateProps
方法,由 React Native 在组件的属性变化时调用;layoutSubviews
方法,描述了自定义视图需要如何布局;webView:didFinishNavigation:
方法,用于处理WKWebView
完成加载页面时需要执行的操作;urlIsValid:(std::string)propString
方法检查接收到的 URL 属性是否有效;eventEmitter
方法,用于检索强类型的eventEmitter
实例;componentDescriptorProvider
方法,返回由 Codegen 生成的ComponentDescriptor
;WebViewCls
方法,用于在应用中注册RCTWebView
。
AppDelegate.mm
最后,可以在应用中注册组件。更新 AppDelegate.mm
文件,使应用意识到自定义的 WebView 组件:
#import "AppDelegate.h"
#import <React/RCTBundleURLProvider.h>
#import <React/RCTBridge+Private.h>
#import "RCTWebView.h"
@implementation AppDelegate
// ...
- (NSDictionary<NSString *,Class<RCTComponentViewProtocol>> *)thirdPartyFabricComponents
{
NSMutableDictionary * dictionary = [super thirdPartyFabricComponents].mutableCopy;
dictionary[@"CustomWebView"] = [RCTWebView class];
return dictionary;
}
@end
这段代码通过获取来自其他来源(如第三方库)的第三方组件字典的可变副本,重写了 thirdPartyFabricComponents
方法。
然后,向字典中添加一个条目,条目名称与 Codegen 规范文件中使用的名称相同。这样,当 React 需要加载名称 CustomWebView
的组件时,React Native 将实例化 RCTWebView
。
最后,返回新的字典。
我们意识到 iOS 存在一些问题,当使用自定义 iOS 组件构建应用时会出现问题。
- 组件需要访问
yoga/style/Style.h
头文件,但当前应用无法访问。为此,将$(PODS_ROOT)/Headers/Private/Yoga
路径添加到应用的头文件搜索路径构建设置中。 - Codegen 在
RCTThirdPartyFabricComponentsProvider
中生成了一行不需要生成的代码。删除RCTThirdPartyFabricComponentsProvider.h
和RCTThirdPartyFabricComponentsProvider.mm
文件中带有WebViewCls
符号的行。
我们已经修复了这些问题,它们将在 React Native 0.76.1 中发布。
3. 使用你的原生组件
最后,你可以在应用中使用该组件。更新你的生成 App.tsx
文件:
import React from 'react';
import {Alert, StyleSheet, View} from 'react-native';
import WebView from './specs/WebViewNativeComponent';
function App(): React.JSX.Element {
return (
<View style={styles.container}>
<WebView
sourceURL="https://react.dev/"
style={styles.webview}
onScriptLoaded={() => {
Alert.alert('Page Loaded');
}}
/>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
alignContent: 'center',
},
webview: {
width: '100%',
height: '100%',
},
});
export default App;
该代码创建了一个使用我们创建的 WebView
组件来加载 react.dev
网站的应用。
该应用还显示了一个当网页加载完成时弹出的警告。
5. 运行应用使用 WebView 组件
- Android
- iOS
yarn run android
yarn run ios
Android | iOS |
---|---|
![]() | ![]() |