集成到现有原生应用
如果你正准备从头开始制作一个新的应用,那么 React Native 会是个非常好的选择。但如果你只想给现有的原生应用中添加一两个视图或是业务流程,React Native 也同样不在话下。只需简单几步,你就可以给原有应用加上新的基于 React Native 的特性、画面和视图等。
具体的步骤根据你所开发的目标平台不同而不同。
译注:本文档可能更新不够及时,不能保证适用于最新版本,欢迎了解的朋友使用页面底部的编辑链接帮忙改进此文档。一个实用的建议是可以使用
npx react-native init NewProject
创建一个最新版本的纯 RN 项目,去参考其 Podfile 或是 gradle 等的配置,以它们为准。
- Android (Java & Kotlin)
- iOS (Objective-C and Swift)
核心概念
把 React Native 组件集成到 Android 应用中有如下几个主要步骤:
- 配置好项目结构。
- 安装必要的 JavaScript 依赖。
- 在 Gradle 中配置 React Native 依赖。
- 创建 ts 文件,编写 React Native 组件的 ts 代码。
- 使用 ReactActivity 来把 React Native 集成到你的 Android 项目代码中。
- 运行 Metro 服务,验证集成结果。
使用社区模板
在跟随本指南时,我们建议你使用 React Native Community Template 作为参考。模板包含一个 精简的 Android app 并且可以帮助你理解如何将 React Native 集成到现有的 Android 应用中。
开发环境准备
首先按照开发环境搭建教程来安装 React Native 在 Android 平台上所需的一切依赖软件。
1. 配置项目目录结构
首先创建一个空目录用于存放 React Native 项目,然后在其中创建一个/android
子目录,把你现有的 Android 项目拷贝到/android
子目录中。
2. 安装 JavaScript 依赖包
在根目录下运行以下命令:
curl -O https://raw.githubusercontent.com/react-native-community/template/refs/heads/0.75-stable/template/package.json
这将把 React Native 社区模板 中的 package.json
文件复制到你的项目中。
接下来我们使用 yarn 或 npm(两者都是 node 的包管理器)来安装必要的模块。请打开一个终端/命令提示行,进入到项目目录中(即包含有 package.json 文件的目录),然后运行下列命令来安装:
- npm
- Yarn
npm install
yarn install
所有 JavaScript 依赖模块都会被安装到项目根目录下的node_modules/
目录中(这个目录我们原则上不复制、不移动、不修改、不上传,随用随装)。
把node_modules/
目录记录到.gitignore
文件中(即不上传到版本控制系统,只保留在本地)。可以参考 React Native 社区模板 中的.gitignore
文件。
把 React Native 添加到你的应用中
配置 Gradle
React Native 使用 React Native Gradle Plugin 来配置您的依赖项和项目设置。
首先,让我们通过添加以下行来编辑您的settings.gradle
文件:
(请参考 社区模板):
// 此处配置用于自动链接第三方原生库的 React Native Gradle 插件
pluginManagement { includeBuild("../node_modules/@react-native/gradle-plugin") }
plugins { id("com.facebook.react.settings") }
extensions.configure(com.facebook.react.ReactSettingsExtension){ ex -> ex.autolinkLibrariesFromCommand() }
// 如果使用 .gradle.kts 文件:
// extensions.configure<com.facebook.react.ReactSettingsExtension> { autolinkLibrariesFromCommand() }
includeBuild("../node_modules/@react-native/gradle-plugin")
// 在这里引入你已有的其他 Gradle 模块。
// include(":app")
然后你需要打开顶层的 build.gradle
文件并添加这一行:
buildscript {
repositories {
google()
mavenCentral()
}
dependencies {
classpath("com.android.tools.build:gradle:7.3.1")
+ classpath("com.facebook.react:react-native-gradle-plugin")
}
}
这将确保 React Native Gradle Plugin 在您的项目中可用。
最后,在 app/build.gradle
文件中添加以下行(注意它的路径不同于上面,这次是 app/build.gradle
):
apply plugin: "com.android.application"
+apply plugin: "com.facebook.react"
repositories {
mavenCentral()
}
dependencies {
// Other dependencies here
+ // 注:我们故意不在这里指定版本号,因为 React Native Gradle Plugin 会自动处理它。
+ // 如果您不使用 React Native Gradle Plugin,则必须手动指定版本。
+ implementation "com.facebook.react:react-android"
+ implementation "com.facebook.react:hermes-android"
}
+react {
+ // 启用自动链接需要添加以下行,参考: https://github.com/react-native-community/cli/blob/master/docs/autolinking.md
+ autolinkLibrariesWithApp()
+}
最后,打开应用的 gradle.properties
文件并添加以下行(请参考 社区模板):
+reactNativeArchitectures=armeabi-v7a,arm64-v8a,x86,x86_64
+newArchEnabled=true
+hermesEnabled=true
配置权限
接着,在 AndroidManifest.xml
清单文件中声明网络权限:
<manifest xmlns:android="http://schemas.android.com/apk/res/android">
+ <uses-permission android:name="android.permission.INTERNET" />
<application
android:name=".MainApplication">
</application>
</manifest>
然后你需要在 AndroidManifest.xml
中启用 允许明文传输 (在src/debug/AndroidManifest.xml
中):
从 Android 9 (API level 28)开始,默认情况下明文传输(http 接口)是禁用的,只能访问 https 接口。这将阻止应用程序连接到Metro bundler。下面的更改允许调试版本中的明文通信。
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<application
+ android:usesCleartextTraffic="true"
+ tools:targetApi="28"
/>
</manifest>
同样可以参考社区模板的 AndroidManifest.xml 文件:main 和 debug
如果希望在正式打包后也能继续访问 http 接口,则需要在src/main/AndroidManifest.xml
中也添加这一选项。
要了解有关网络安全配置和明文通信策略的更多信息,请参阅此链接。
代码集成
现在我们将修改原生 Android 应用程序以集成 React Native。
React Native 组件
我们首先要写的是"High Score"(得分排行榜)的 JavaScript 端的代码。
创建一个index.js
文件
首先在项目根目录中创建一个空的index.js
文件。
index.js
是 React Native 应用在 Android 上的入口文件。而且它是不可或缺的!它可以是个很简单的文件,简单到可以只包含一行require/import
导入语句。
本教程的index.js
文件应该如下所示(请参考 社区模板):
import {AppRegistry} from 'react-native';
import App from './App';
AppRegistry.registerComponent('HelloWorld', () => App);
创建一个 App.tsx
文件
下面我们创建一个 App.tsx
文件。这是一个 TypeScript 文件,可以包含 JSX 表达式。它包含了我们将在 Android 应用中集成的根 React Native 组件(请参考 社区模板):
import React from 'react';
import {
SafeAreaView,
ScrollView,
StatusBar,
StyleSheet,
Text,
useColorScheme,
View,
} from 'react-native';
import {
Colors,
DebugInstructions,
Header,
ReloadInstructions,
} from 'react-native/Libraries/NewAppScreen';
function App(): React.JSX.Element {
const isDarkMode = useColorScheme() === 'dark';
const backgroundStyle = {
backgroundColor: isDarkMode ? Colors.darker : Colors.lighter,
};
return (
<SafeAreaView style={backgroundStyle}>
<StatusBar
barStyle={isDarkMode ? 'light-content' : 'dark-content'}
backgroundColor={backgroundStyle.backgroundColor}
/>
<ScrollView
contentInsetAdjustmentBehavior="automatic"
style={backgroundStyle}>
<Header />
<View
style={{
backgroundColor: isDarkMode
? Colors.black
: Colors.white,
padding: 24,
}}>
<Text style={styles.title}>Step One</Text>
<Text>
Edit <Text style={styles.bold}>App.tsx</Text> to
change this screen and see your edits.
</Text>
<Text style={styles.title}>See your changes</Text>
<ReloadInstructions />
<Text style={styles.title}>Debug</Text>
<DebugInstructions />
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
title: {
fontSize: 24,
fontWeight: '600',
},
bold: {
fontWeight: '700',
},
});
export default App;
5. Integrating with your Android code
We now need to add some native code in order to start the React Native runtime and tell it to render our React components.
Updating your Application class
First, we need to update your Application
class to properly initialize React Native as follows:
- Java
- Kotlin
package <your-package-here>;
import android.app.Application;
+import com.facebook.react.PackageList;
+import com.facebook.react.ReactApplication;
+import com.facebook.react.ReactHost;
+import com.facebook.react.ReactNativeHost;
+import com.facebook.react.ReactPackage;
+import com.facebook.react.defaults.DefaultNewArchitectureEntryPoint;
+import com.facebook.react.defaults.DefaultReactHost;
+import com.facebook.react.defaults.DefaultReactNativeHost;
+import com.facebook.soloader.SoLoader;
+import com.facebook.react.soloader.OpenSourceMergedSoMapping
+import java.util.List;
-class MainApplication extends Application {
+class MainApplication extends Application implements ReactApplication {
+ @Override
+ public ReactNativeHost getReactNativeHost() {
+ return new DefaultReactNativeHost(this) {
+ @Override
+ protected List<ReactPackage> getPackages() { return new PackageList(this).getPackages(); }
+ @Override
+ protected String getJSMainModuleName() { return "index"; }
+ @Override
+ public boolean getUseDeveloperSupport() { return BuildConfig.DEBUG; }
+ @Override
+ protected boolean isNewArchEnabled() { return BuildConfig.IS_NEW_ARCHITECTURE_ENABLED; }
+ @Override
+ protected Boolean isHermesEnabled() { return BuildConfig.IS_HERMES_ENABLED; }
+ };
+ }
+ @Override
+ public ReactHost getReactHost() {
+ return DefaultReactHost.getDefaultReactHost(getApplicationContext(), getReactNativeHost());
+ }
@Override
public void onCreate() {
super.onCreate();
+ SoLoader.init(this, OpenSourceMergedSoMapping);
+ if (BuildConfig.IS_NEW_ARCHITECTURE_ENABLED) {
+ DefaultNewArchitectureEntryPoint.load();
+ }
}
}
// package <your-package-here>
import android.app.Application
+import com.facebook.react.PackageList
+import com.facebook.react.ReactApplication
+import com.facebook.react.ReactHost
+import com.facebook.react.ReactNativeHost
+import com.facebook.react.ReactPackage
+import com.facebook.react.defaults.DefaultNewArchitectureEntryPoint.load
+import com.facebook.react.defaults.DefaultReactHost.getDefaultReactHost
+import com.facebook.react.defaults.DefaultReactNativeHost
+import com.facebook.soloader.SoLoader
+import com.facebook.react.soloader.OpenSourceMergedSoMapping
-class MainApplication : Application() {
+class MainApplication : Application(), ReactApplication {
+ override val reactNativeHost: ReactNativeHost =
+ object : DefaultReactNativeHost(this) {
+ override fun getPackages(): List<ReactPackage> = PackageList(this).packages
+ override fun getJSMainModuleName(): String = "index"
+ override fun getUseDeveloperSupport(): Boolean = BuildConfig.DEBUG
+ override val isNewArchEnabled: Boolean = BuildConfig.IS_NEW_ARCHITECTURE_ENABLED
+ override val isHermesEnabled: Boolean = BuildConfig.IS_HERMES_ENABLED
+ }
+ override val reactHost: ReactHost
+ get() = getDefaultReactHost(applicationContext, reactNativeHost)
override fun onCreate() {
super.onCreate()
+ SoLoader.init(this, OpenSourceMergedSoMapping)
+ if (BuildConfig.IS_NEW_ARCHITECTURE_ENABLED) {
+ load()
+ }
}
}
As usual, here the MainApplication.kt Community template file as reference
Creating a ReactActivity
Finally, we need to create a new Activity
that will extend ReactActivity
and host the React Native code. This activity will be responsible for starting the React Native runtime and rendering the React component.
- Java
- Kotlin
// package <your-package-here>;
import com.facebook.react.ReactActivity;
import com.facebook.react.ReactActivityDelegate;
import com.facebook.react.defaults.DefaultNewArchitectureEntryPoint;
import com.facebook.react.defaults.DefaultReactActivityDelegate;
public class MyReactActivity extends ReactActivity {
@Override
protected String getMainComponentName() {
return "HelloWorld";
}
@Override
protected ReactActivityDelegate createReactActivityDelegate() {
return new DefaultReactActivityDelegate(this, getMainComponentName(), DefaultNewArchitectureEntryPoint.getFabricEnabled());
}
}
// package <your-package-here>
import com.facebook.react.ReactActivity
import com.facebook.react.ReactActivityDelegate
import com.facebook.react.defaults.DefaultNewArchitectureEntryPoint.fabricEnabled
import com.facebook.react.defaults.DefaultReactActivityDelegate
class MyReactActivity : ReactActivity() {
override fun getMainComponentName(): String = "HelloWorld"
override fun createReactActivityDelegate(): ReactActivityDelegate =
DefaultReactActivityDelegate(this, mainComponentName, fabricEnabled)
}
As usual, here the MainActivity.kt Community template file as reference
Whenever you create a new Activity, you need to add it to your AndroidManifest.xml
file. You also need set the theme of MyReactActivity
to Theme.AppCompat.Light.NoActionBar
(or to any non-ActionBar theme) as otherwise your application will render an ActionBar on top of your React Native screen:
<manifest xmlns:android="http://schemas.android.com/apk/res/android">
<uses-permission android:name="android.permission.INTERNET" />
<application
android:name=".MainApplication">
+ <activity
+ android:name=".MyReactActivity"
+ android:label="@string/app_name"
+ android:theme="@style/Theme.AppCompat.Light.NoActionBar">
+ </activity>
</application>
</manifest>
Now your activity is ready to run some JavaScript code.
6. Test your integration
You have completed all the basic steps to integrate React Native with your application. Now we will start the Metro bundler to build your TypeScript application code into a bundle. Metro's HTTP server shares the bundle from localhost
on your developer environment to a simulator or device. This allows for hot reloading.
First, you need to create a metro.config.js
file in the root of your project as follows:
const {getDefaultConfig} = require('@react-native/metro-config');
module.exports = getDefaultConfig(__dirname);
You can checkout the metro.config.js file from the Community template file as reference.
Once you have the config file in place, you can run the bundler. Run the following command in the root directory of your project:
- npm
- Yarn
npm start
yarn start
Now build and run your Android app as normal.
Once you reach your React-powered Activity inside the app, it should load the JavaScript code from the development server and display:
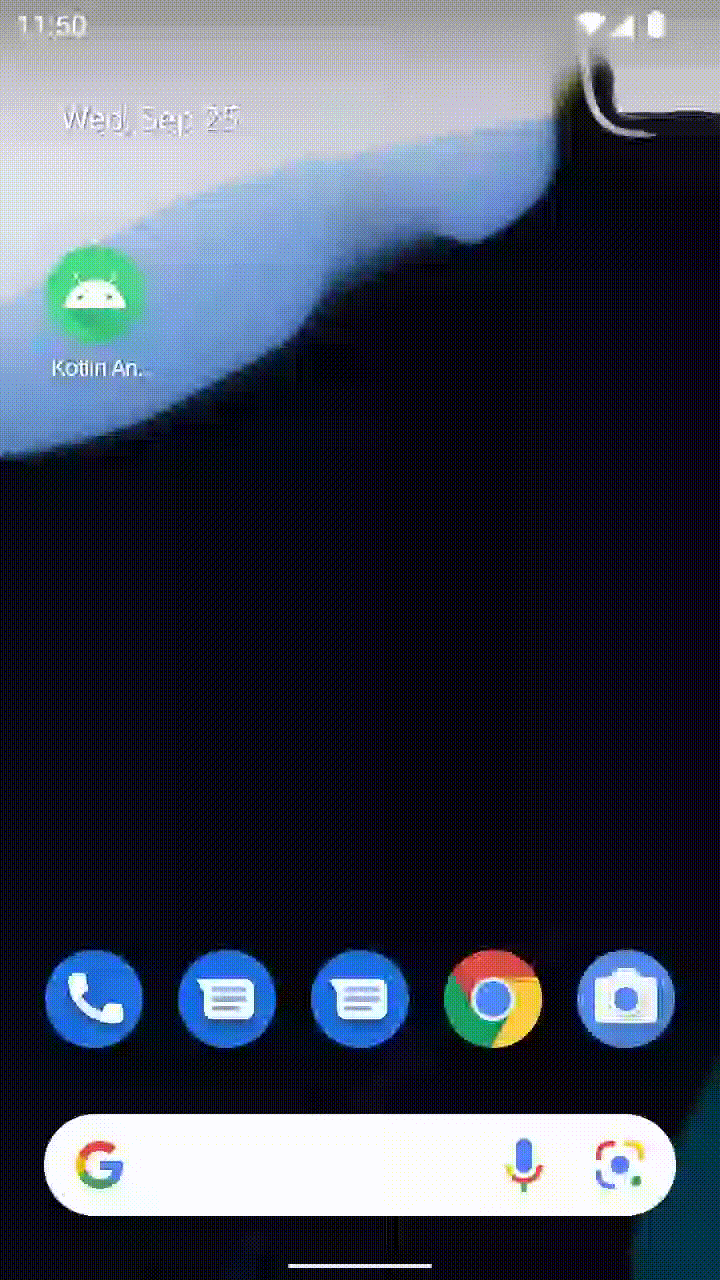
Creating a release build in Android Studio
You can use Android Studio to create your release builds too! It’s as quick as creating release builds of your previously-existing native Android app.
The React Native Gradle Plugin will take care of bundling the JS code inside your APK/App Bundle.
If you're not using Android Studio, you can create a release build with:
cd android
# For a Release APK
./gradlew :app:assembleRelease
# For a Release AAB
./gradlew :app:bundleRelease
Now what?
At this point you can continue developing your app as usual. Refer to our debugging and deployment docs to learn more about working with React Native.
关键概念
将 React Native 组件集成到 iOS 应用程序中的关键步骤是:
- 设置正确的目录结构。
- 安装必要的 NPM 依赖项。
- 在 Podfile 配置中添加 React Native。
- 为你的第一个 React Native 屏幕编写 TypeScript 代码。
- 使用
RCTRootView
将 React Native 与你的 iOS 代码集成。 - 通过运行打包器并查看应用程序运行情况来测试你的集成。
使用社区模板
在您遵循本指南时,我们建议您参考React Native 社区模板。该模板包含一个最小化的 iOS 应用,将帮助您理解如何将 React Native 集成到现有的 iOS 应用中。
准备工作
请按照设置开发环境指南指南来配置您的开发环境,以便构建 iOS 平台的 React Native 应用。
本指南还假设您熟悉 iOS 开发的基础知识,如创建UIViewController
和编辑Podfile
文件。
1. 设置目录结构
为确保顺利体验,请为您的集成 React Native 项目创建一个新文件夹,然后将现有的 iOS 项目移动到 /ios
子文件夹中。
2. 安装 NPM 依赖
进入根目录并运行以下命令:
curl -O https://raw.githubusercontent.com/react-native-community/template/refs/heads/0.78-stable/template/package.json
这将从社区模板 复制 package.json
文件到您的项目中。
接下来,运行以下命令安装 NPM 包:
- npm
- Yarn
npm install
yarn install
安装过程创建了一个新的 node_modules
文件夹。该文件夹存储了构建项目所需的 JavaScript 依赖项。
将 node_modules/
添加到您的 .gitignore
文件中(社区默认文件)。
3. 安装开发工具
Xcode 命令行工具
安装命令行工具。选择 Xcode 菜单中的 Settings...(或 Preferences...)。转到位置面板,并安装工具,方法是选择命令行工具下拉菜单中最新的版本。
CocoaPods
CocoaPods 是 iOS 和 macOS 开发的包管理工具。我们使用它将实际的 React Native 框架代码添加到您的当前项目中。
我们建议使用 Homebrew 安装 CocoaPods:
brew install cocoapods
4. 将 React Native 添加到您的应用
配置 CocoaPods
要配置 CocoaPods,我们需要两个文件:
- 一个 Gemfile 文件,定义了我们需要的 Ruby 依赖项。
- 一个 Podfile 文件,定义了如何正确安装我们的依赖项。
对于 Gemfile,请进入您的项目根目录并运行以下命令:
curl -O https://raw.githubusercontent.com/react-native-community/template/refs/heads/0.78-stable/template/Gemfile
这将下载 Gemfile 文件。
对于 Podfile,请进入您的项目 ios
文件夹并运行以下命令:
curl -O https://raw.githubusercontent.com/react-native-community/template/refs/heads/0.78-stable/template/ios/Podfile
请使用社区模板 作为 Gemfile 和 Podfile 的参考。
请记住更改 Podfile 中的这行,以匹配您的应用名称。
现在,我们需要运行一些额外的命令来安装 Ruby Gem 和 Pods。
进入 ios
文件夹并运行以下命令:
bundle install
bundle exec pod install
第一个命令将安装 Ruby 依赖项,第二个命令将实际将 React Native 代码集成到您的应用程序中,以便您的 iOS 文件可以导入 React Native 头文件。
5. 编写 TypeScript 代码
现在我们将修改原生 iOS 应用程序以集成 React Native。
我们将编写的第一个代码片段是实际的 React Native 代码,该代码将集成到我们的应用程序中。
创建一个 index.js
文件
首先,在 React Native 项目的根目录中创建一个空的 index.js
文件。
index.js
是 React Native 应用程序的起点,并且总是需要。它可以是一个小文件,该文件 import
其他文件,这些文件是您的 React Native 组件或应用程序的一部分,或者它可以包含所有需要的代码。
我们的 index.js
文件应如下所示(社区模板文件 作为参考):
import {AppRegistry} from 'react-native';
import App from './App';
AppRegistry.registerComponent('HelloWorld', () => App);
创建一个 App.tsx
文件
让我们创建一个 App.tsx
文件。这是一个 TypeScript 文件,可以包含 JSX 表达式。它包含我们要集成到 iOS 应用程序中的根 React Native 组件(链接):
import React from 'react';
import {
SafeAreaView,
ScrollView,
StatusBar,
StyleSheet,
Text,
useColorScheme,
View,
} from 'react-native';
import {
Colors,
DebugInstructions,
Header,
ReloadInstructions,
} from 'react-native/Libraries/NewAppScreen';
function App(): React.JSX.Element {
const isDarkMode = useColorScheme() === 'dark';
const backgroundStyle = {
backgroundColor: isDarkMode ? Colors.darker : Colors.lighter,
};
return (
<SafeAreaView style={backgroundStyle}>
<StatusBar
barStyle={isDarkMode ? 'light-content' : 'dark-content'}
backgroundColor={backgroundStyle.backgroundColor}
/>
<ScrollView
contentInsetAdjustmentBehavior="automatic"
style={backgroundStyle}>
<Header />
<View
style={{
backgroundColor: isDarkMode
? Colors.black
: Colors.white,
padding: 24,
}}>
<Text style={styles.title}>Step One</Text>
<Text>
Edit <Text style={styles.bold}>App.tsx</Text> to
change this screen and see your edits.
</Text>
<Text style={styles.title}>See your changes</Text>
<ReloadInstructions />
<Text style={styles.title}>Debug</Text>
<DebugInstructions />
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
title: {
fontSize: 24,
fontWeight: '600',
},
bold: {
fontWeight: '700',
},
});
export default App;
社区模板文件 作为参考
5. 与 iOS 代码集成
我们现在需要添加一些原生代码,以便启动 React Native 运行时并告诉它渲染我们的 React 组件。
准备工作
React Native 的初始化与 iOS 应用的其他部分无关。
React Native 可以通过一个名为 RCTReactNativeFactory
的类来初始化,该类负责处理 React Native 的生命周期。
一旦类被初始化,您可以启动一个 React Native 视图,提供一个 UIWindow
对象,或者您可以要求工厂生成一个 UIView
,您可以在任何 UIViewController
中加载它。
在以下示例中,我们将创建一个可以加载 React Native 视图的 ViewController。
创建一个 ReactViewController
从模板创建一个新文件 (⌘+N) 并选择 Cocoa Touch Class 模板。
确保选择 UIViewController
作为 "Subclass of" 字段。
- ObjectiveC
- Swift
现在打开 ReactViewController.m
文件并应用以下更改
#import "ReactViewController.h"
+#import <React/RCTBundleURLProvider.h>
+#import <RCTReactNativeFactory.h>
+#import <RCTDefaultReactNativeFactoryDelegate.h>
+#import <RCTAppDependencyProvider.h>
@interface ReactViewController ()
@end
+@interface ReactNativeFactoryDelegate: RCTDefaultReactNativeFactoryDelegate
+@end
-@implementation ReactViewController
+@implementation ReactViewController {
+ RCTReactNativeFactory *_factory;
+ id<RCTReactNativeFactoryDelegate> _factoryDelegate;
+}
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view.
+ _factoryDelegate = [ReactNativeFactoryDelegate new];
+ _factoryDelegate.dependencyProvider = [RCTAppDependencyProvider new];
+ _factory = [[RCTReactNativeFactory alloc] initWithDelegate:_factoryDelegate];
+ self.view = [_factory.rootViewFactory viewWithModuleName:@"HelloWorld"];
}
@end
+@implementation ReactNativeFactoryDelegate
+
+- (NSURL *)sourceURLForBridge:(RCTBridge *)bridge
+{
+ return [self bundleURL];
+}
+
+- (NSURL *)bundleURL
+{
+#if DEBUG
+ return [RCTBundleURLProvider.sharedSettings jsBundleURLForBundleRoot:@"index"];
+#else
+ return [NSBundle.mainBundle URLForResource:@"main" withExtension:@"jsbundle"];
+#endif
+}
@end
现在打开 ReactViewController.swift
文件并应用以下更改
import UIKit
+import React
+import React_RCTAppDelegate
+import ReactAppDependencyProvider
class ReactViewController: UIViewController {
+ var reactNativeFactory: RCTReactNativeFactory?
+ var reactNativeFactoryDelegate: RCTReactNativeFactoryDelegate?
override func viewDidLoad() {
super.viewDidLoad()
+ reactNativeFactoryDelegate = ReactNativeDelegate()
+ reactNativeFactoryDelegate!.dependencyProvider = RCTAppDependencyProvider()
+ reactNativeFactory = RCTReactNativeFactory(delegate: reactNativeFactoryDelegate!)
+ view = reactNativeFactory!.rootViewFactory.view(withModuleName: "HelloWorld")
}
}
+class ReactNativeDelegate: RCTDefaultReactNativeFactoryDelegate {
+ override func sourceURL(for bridge: RCTBridge) -> URL? {
+ self.bundleURL()
+ }
+
+ override func bundleURL() -> URL? {
+ #if DEBUG
+ RCTBundleURLProvider.sharedSettings().jsBundleURL(forBundleRoot: "index")
+ #else
+ Bundle.main.url(forResource: "main", withExtension: "jsbundle")
+ #endif
+ }
+
+}
在 rootViewController 中展示 React Native 视图
最后,我们可以展示我们的 React Native 视图。为此,我们需要一个可以承载视图的新视图控制器,我们可以在其中加载 JS 内容。
- 从 Xcode 中,创建一个新
UIViewController
(我们称之为ReactViewController
)。 - 让初始
ViewController
展示ReactViewController
。有几种方法可以做到这一点,具体取决于您的应用程序。对于此示例,我们假设您有一个按钮,用于模态展示 React Native。
- ObjectiveC
- Swift
#import "ViewController.h"
+#import "ReactViewController.h"
@interface ViewController ()
@end
- @implementation ViewController
+@implementation ViewController {
+ ReactViewController *reactViewController;
+}
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view.
self.view.backgroundColor = UIColor.systemBackgroundColor;
+ UIButton *button = [UIButton new];
+ [button setTitle:@"Open React Native" forState:UIControlStateNormal];
+ [button setTitleColor:UIColor.systemBlueColor forState:UIControlStateNormal];
+ [button setTitleColor:UIColor.blueColor forState:UIControlStateHighlighted];
+ [button addTarget:self action:@selector(presentReactNative) forControlEvents:UIControlEventTouchUpInside];
+ [self.view addSubview:button];
+ button.translatesAutoresizingMaskIntoConstraints = NO;
+ [NSLayoutConstraint activateConstraints:@[
+ [button.leadingAnchor constraintEqualToAnchor:self.view.leadingAnchor],
+ [button.trailingAnchor constraintEqualToAnchor:self.view.trailingAnchor],
+ [button.centerYAnchor constraintEqualToAnchor:self.view.centerYAnchor],
+ [button.centerXAnchor constraintEqualToAnchor:self.view.centerXAnchor],
+ ]];
}
+- (void)presentReactNative
+{
+ if (reactViewController == NULL) {
+ reactViewController = [ReactViewController new];
+ }
+ [self presentViewController:reactViewController animated:YES completion:nil];
+}
@end
import UIKit
class ViewController: UIViewController {
+ var reactViewController: ReactViewController?
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
self.view.backgroundColor = .systemBackground
+ let button = UIButton()
+ button.setTitle("Open React Native", for: .normal)
+ button.setTitleColor(.systemBlue, for: .normal)
+ button.setTitleColor(.blue, for: .highlighted)
+ button.addAction(UIAction { [weak self] _ in
+ guard let self else { return }
+ if reactViewController == nil {
+ reactViewController = ReactViewController()
+ }
+ present(reactViewController!, animated: true)
+ }, for: .touchUpInside)
+ self.view.addSubview(button)
+
+ button.translatesAutoresizingMaskIntoConstraints = false
+ NSLayoutConstraint.activate([
+ button.leadingAnchor.constraint(equalTo: self.view.leadingAnchor),
+ button.trailingAnchor.constraint(equalTo: self.view.trailingAnchor),
+ button.centerXAnchor.constraint(equalTo: self.view.centerXAnchor),
+ button.centerYAnchor.constraint(equalTo: self.view.centerYAnchor),
+ ])
}
}
确保禁用沙盒脚本。为此,在 Xcode 中,点击您的应用,然后点击构建设置。过滤脚本并设置 User Script Sandboxing
为 NO
。这一步是为了在调试和发布版本之间正确切换 Hermes 引擎。
;
最后,确保在您的 Info.plist
文件中添加 UIViewControllerBasedStatusBarAppearance
键,值为 NO
。
6. 测试您的集成
您已经完成了将 React Native 与您的应用程序集成所需的所有基本步骤。现在我们将启动 Metro bundler 来构建您的 TypeScript 应用程序代码。Metro 的 HTTP 服务器从您的开发环境共享 bundle 到模拟器或设备。这允许 热重载。
首先,您需要在项目根目录中创建一个 metro.config.js
文件,如下所示:
const {getDefaultConfig} = require('@react-native/metro-config');
module.exports = getDefaultConfig(__dirname);
您可以查看社区模板文件 作为参考。
Then, you need to create a .watchmanconfig
file in the root of your project. The file must contain an empty json object:
echo {} > .watchmanconfig
一旦您有了配置文件,您可以运行 bundler。在项目根目录下运行以下命令:
- npm
- Yarn
npm start
yarn start
现在,像往常一样构建和运行您的 iOS 应用。
一旦您到达您的 React 驱动的 Activity 中,它应该从开发服务器加载 JavaScript 代码并显示:
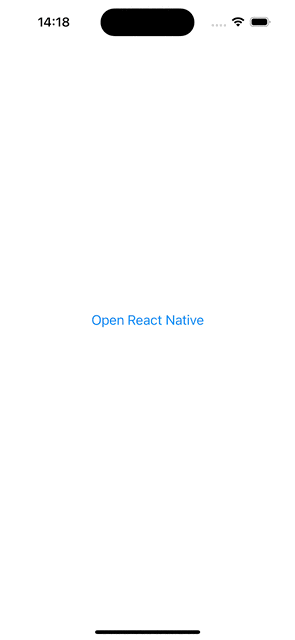
在 Xcode 中创建发布版本
您可以使用 Xcode 创建您的发布版本!唯一的额外步骤是添加一个脚本,当应用程序构建时,将您的 JS 和图像打包到 iOS 应用程序中。
- 在 Xcode 中,选择您的应用
- 点击
Build Phases
- 点击左上角的
+
并选择New Run Script Phase
- 点击
Run Script
行并重命名脚本为Bundle React Native code and images
- 在文本框中粘贴以下脚本
set -e
WITH_ENVIRONMENT="$REACT_NATIVE_PATH/scripts/xcode/with-environment.sh"
REACT_NATIVE_XCODE="$REACT_NATIVE_PATH/scripts/react-native-xcode.sh"
/bin/sh -c "$WITH_ENVIRONMENT $REACT_NATIVE_XCODE"
- 将脚本拖放到名为
[CP] Embed Pods Frameworks
的脚本之前。
现在,如果您为发布版本构建您的应用,它将按预期工作。
7. 将初始属性传递给 React Native 视图
在某些情况下,您可能希望从原生应用传递一些信息到 JavaScript。例如,您可能希望传递当前登录用户的用户 ID 和令牌,以便从数据库中检索信息。
这可以通过使用 RCTReactNativeFactory
类的 view(withModuleName:initialProperty)
重载的 initialProperties
参数来实现。以下步骤展示了如何实现。
更新 App.tsx 文件以读取初始属性。
打开 App.tsx
文件并添加以下代码:
import {
Colors,
DebugInstructions,
Header,
ReloadInstructions,
} from 'react-native/Libraries/NewAppScreen';
-function App(): React.JSX.Element {
+function App(props): React.JSX.Element {
const isDarkMode = useColorScheme() === 'dark';
const backgroundStyle = {
backgroundColor: isDarkMode ? Colors.darker : Colors.lighter,
};
return (
<SafeAreaView style={backgroundStyle}>
<StatusBar
barStyle={isDarkMode ? 'light-content' : 'dark-content'}
backgroundColor={backgroundStyle.backgroundColor}
/>
<ScrollView
contentInsetAdjustmentBehavior="automatic"
style={backgroundStyle}>
<Header />
- <View
- style={{
- backgroundColor: isDarkMode
- ? Colors.black
- : Colors.white,
- padding: 24,
- }}>
- <Text style={styles.title}>Step One</Text>
- <Text>
- Edit <Text style={styles.bold}>App.tsx</Text> to
- change this screen and see your edits.
- </Text>
- <Text style={styles.title}>See your changes</Text>
- <ReloadInstructions />
- <Text style={styles.title}>Debug</Text>
- <DebugInstructions />
+ <Text style={styles.title}>UserID: {props.userID}</Text>
+ <Text style={styles.title}>Token: {props.token}</Text>
</View>
</ScrollView>
</SafeAreaView>
);
}
const styles = StyleSheet.create({
title: {
fontSize: 24,
fontWeight: '600',
+ marginLeft: 20,
},
bold: {
fontWeight: '700',
},
});
export default App;
这些更改将告诉 React Native 您的 App 组件现在接受一些属性。RCTreactNativeFactory
将负责在组件渲染时将它们传递给它。
更新原生代码以将初始属性传递给 JavaScript。
- ObjectiveC
- Swift
修改 ReactViewController.mm
以将初始属性传递给 JavaScript。
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view.
_factoryDelegate = [ReactNativeFactoryDelegate new];
_factoryDelegate.dependencyProvider = [RCTAppDependencyProvider new];
_factory = [[RCTReactNativeFactory alloc] initWithDelegate:_factoryDelegate];
- self.view = [_factory.rootViewFactory viewWithModuleName:@"HelloWorld"];
+ self.view = [_factory.rootViewFactory viewWithModuleName:@"HelloWorld" initialProperties:@{
+ @"userID": @"12345678",
+ @"token": @"secretToken"
+ }];
}
修改 ReactViewController.swift
以将初始属性传递给 React Native 视图。
override func viewDidLoad() {
super.viewDidLoad()
reactNativeFactoryDelegate = ReactNativeDelegate()
reactNativeFactoryDelegate!.dependencyProvider = RCTAppDependencyProvider()
reactNativeFactory = RCTReactNativeFactory(delegate: reactNativeFactoryDelegate!)
- view = reactNativeFactory!.rootViewFactory.view(withModuleName: "HelloWorld")
+ view = reactNativeFactory!.rootViewFactory.view(withModuleName: "HelloWorld" initialProperties: [
+ "userID": "12345678",
+ "token": "secretToken"
+])
}
}
- 再次运行您的应用。您应该在展示
ReactViewController
后看到以下屏幕:
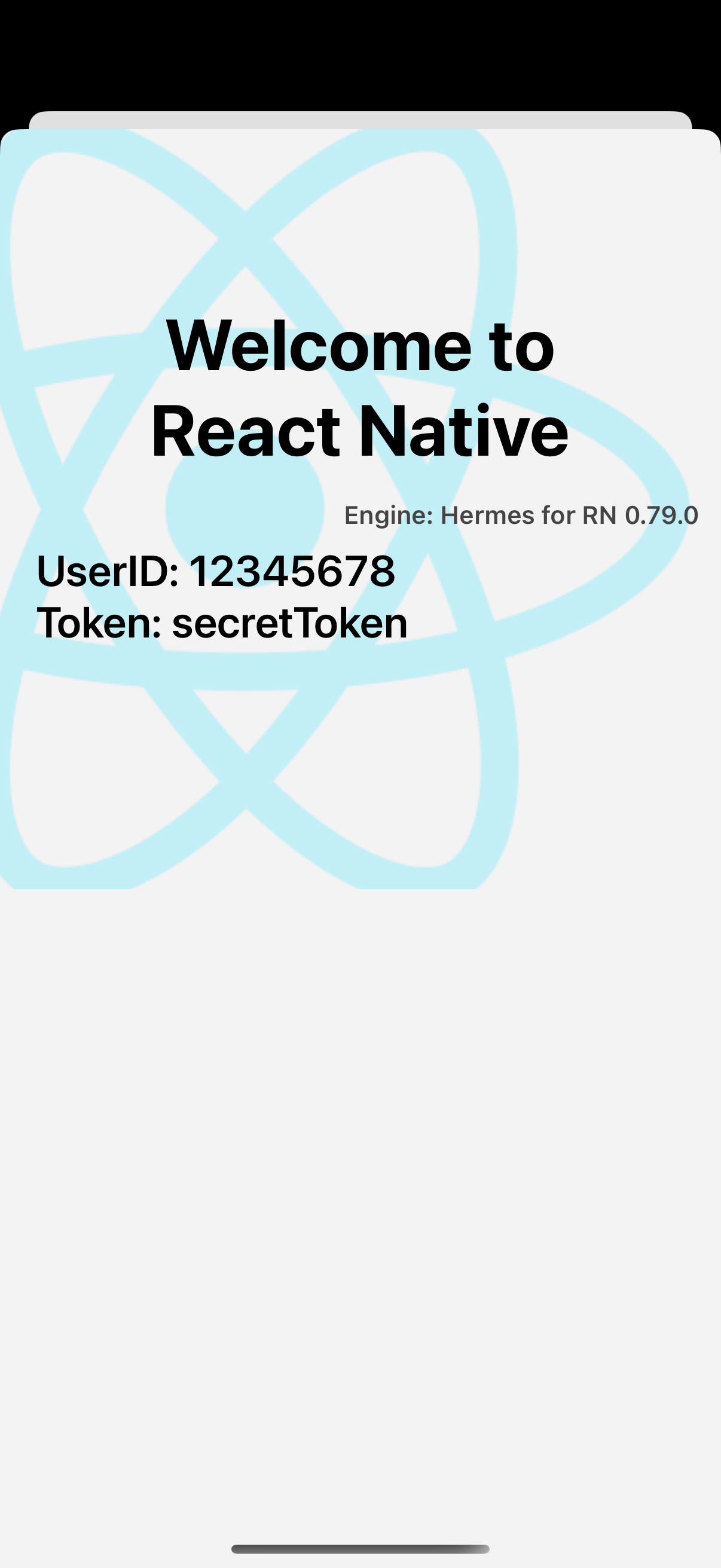
现在呢?
此时,您可以继续像往常一样开发您的应用。请参阅我们的调试和部署文档,了解更多关于使用 React Native 的信息。